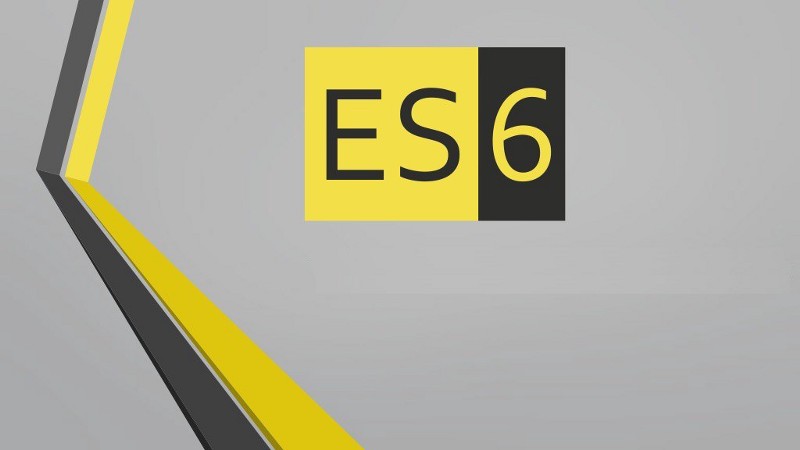
Per chi non lo sapesse ES6 o ufficialmente ECMAScript2015 è l’ultima implementazione del noto linguaggio di programmazione Javascript. Vista la crescente diffusione nell’uso di questo linguaggio e l’ evoluzione che lo ha trasformato da un linguaggio prettamente per il web o meglio un linguaggio che funzionava solo all’interno di un browser web, in un linguaggio general purpose conoscere le nuove e importanti caratteristiche introdotte in quest’ultima versione è una cosa che giova molto a tutti i programmatori: D’altronde chi non ha mai usato javascript in una propria applicazione?
Indice
Un minimo di storia
- 1995: nasce JavaScript ma il suo nome è LiveScript
- 1997: Nasce lo standard ECMAScript
- 1999: Nasce ES3 e IE5 è il browser maggiormente in voga
- 2000–2005: l’uso dell’oggetto XMLHttpRequest, meglio conosciuto come AJAX, che consente comunicazioni asincrone, si diffonde rapidamente con la nascita di applicazioni come Outlook Web Access (2000), Gmail (2004) e Google Maps (2005).
- 2009: Esce ES5 (attualmente la versione più usata e più supportata) con istruzioni come:
forEach
,Object.keys
,Object.create
e lo standard JSON - 2015: Nasce ES6/ECMAScript2015 con tantissime migliorie. Alcune delle più importati saranno descritte di seguito
Adesso vediamo 10 delle nuove caratteristiche introdotte in ES6:
1. Valori di default dei parametri
ES5
function f (x, y, z) { if (x === undefined) x = 0; if (y === undefined) y = 7; if (z === undefined) z = 42; return x + y + z; };
ES6
function f (x=0, y = 7, z = 42) { return x + y + z }
2. Interpolazione di stringhe
ES5
var ws = ' Benvenuto ' + first + ' ' + last + '.' var url = 'http://localhost:8080/api/user/' + id
ES6
var ws= `Benvenuto ${first} ${last}.` var url = `http://localhost:8080/api/user/${id}`
3. Stringhe multilinea
ES5
var poema = 'Nel mezzo del cammin di nostra vita\n\t'+ 'mi ritrovai per una selva oscura,\n\t'+ 'ché la diritta via era smarrita.';
ES6
var poema = 'Nel mezzo del cammin di nostra vita mi ritrovai per una selva oscura, ché la diritta via era smarrita.';
4. Assegnamenti destrutturati
destrutturazione intuitiva e flessibile di oggetti e array in singole variabili durante l’assegnazione.
ES5
var user = getUser(); // user è un oggetto che ha il campi nome, cognome e nickname var nome = user.nome; var cognome = user.cognome; var nick = user.nickname; var list = [ 1, 2, 3 ]; var a = list[0], b = list[2];
ES6
var { nome, cognome, nick} = getUser(); var list = [ 1, 2, 3 ]; var [ a, , b ] = list;
5. Notazione più semplice delle proprietà degli oggetti e dei metodi
ES5
obj = { x: x, y: y }; obj = { foo: function (a, b) { … }, bar: function (x, y) { … }, // quux: no equivalent in ES5 … };
ES6
obj = { x, y }; obj = { foo (a, b) { … }, bar (x, y) { … }, *quux (x, y) { … } }
6. Funzioni freccia
ES5
const squares = arr.map(function (x) { return x * x });
ES6
const squares = arr.map(x => x * x);
7. Promises
ES5
function isGreater (a, b, cb) { var greater = false if(a > b) { greater = true } cb(greater) } isGreater(1, 2, function (result) { if(result) { console.log('greater'); } else { console.log('smaller') } })
ES6
const isGreater = (a, b) => { return new Promise ((resolve, reject) => { if(a > b) { resolve(true) } else { reject(false) } }) } isGreater(1, 2) .then(result => { console.log('greater') }) .catch(result => { console.log('smaller') })
Le Promises di ES6 ci consentono di risolvere (resolve) e respingere (reject) una richiesta. Ogni volta che risolviamo una richiesta chiameremo la callback indicata nel then e ogni volta che rifiuteremo una richiesta chiameremo la callback della catch.
Le Promises ES6 sono migliori delle callback perché ci permettono di distinguere facilmente tra un successo e un errore, quindi non dobbiamo controllare di nuovo le cose nella nostra funzione di callback.
8. Export ed import di moduli
ES5
// Export var myModule = { x: 1, y: function(){ console.log('This is ES5') }} module.exports = myModule; // Import var myModule = require('./myModule');
ES6
//Export const myModule = { x: 1, y: () => { console.log('This is ES5') }} export default myModule; // Import import myModule from './myModule';
9. Classi
Notazione delle Classi più intuitiva in stile OOP e priva di codice boilerplate
Definizione delle Classi
ES5
var Shape = function (id, x, y) { this.id = id; this.move(x, y); }; Shape.prototype.move = function (x, y) { this.x = x; this.y = y; };
ES6
class Shape { constructor (id, x, y) { this.id = id this.move(x, y) } move (x, y) { this.x = x this.y = y } }
Ereditarietà
ES5
var Rectangle = function (id, x, y, width, height) { Shape.call(this, id, x, y); this.width = width; this.height = height; }; Rectangle.prototype = Object.create(Shape.prototype); Rectangle.prototype.constructor = Rectangle; var Circle = function (id, x, y, radius) { Shape.call(this, id, x, y); this.radius = radius; }; Circle.prototype = Object.create(Shape.prototype); Circle.prototype.constructor = Circle;
ES6
class Rectangle extends Shape { constructor (id, x, y, width, height) { super(id, x, y) this.width = width this.height = height } } class Circle extends Shape { constructor (id, x, y, radius) { super(id, x, y) this.radius = radius } }
10. Scope a livello di Blocco
Variabili
ES5
var i, x, y; for (i = 0; i < a.length; i++) { x = a[i]; … } for (i = 0; i < b.length; i++) { y = b[i]; … }
ES6
for (let i = 0; i < a.length; i++) { let x = a[i] … } for (let i = 0; i < b.length; i++) { let y = b[i] … }
Funzioni
ES5
(function () { var foo = function () { return 1; } foo() === 1; (function () { var foo = function () { return 2; } foo() === 2; })(); foo() === 1; })();
ES6
{ function foo () { return 1 } foo() === 1 { function foo () { return 2 } foo() === 2 } foo() === 1 }